Embedded Integration
How It Works
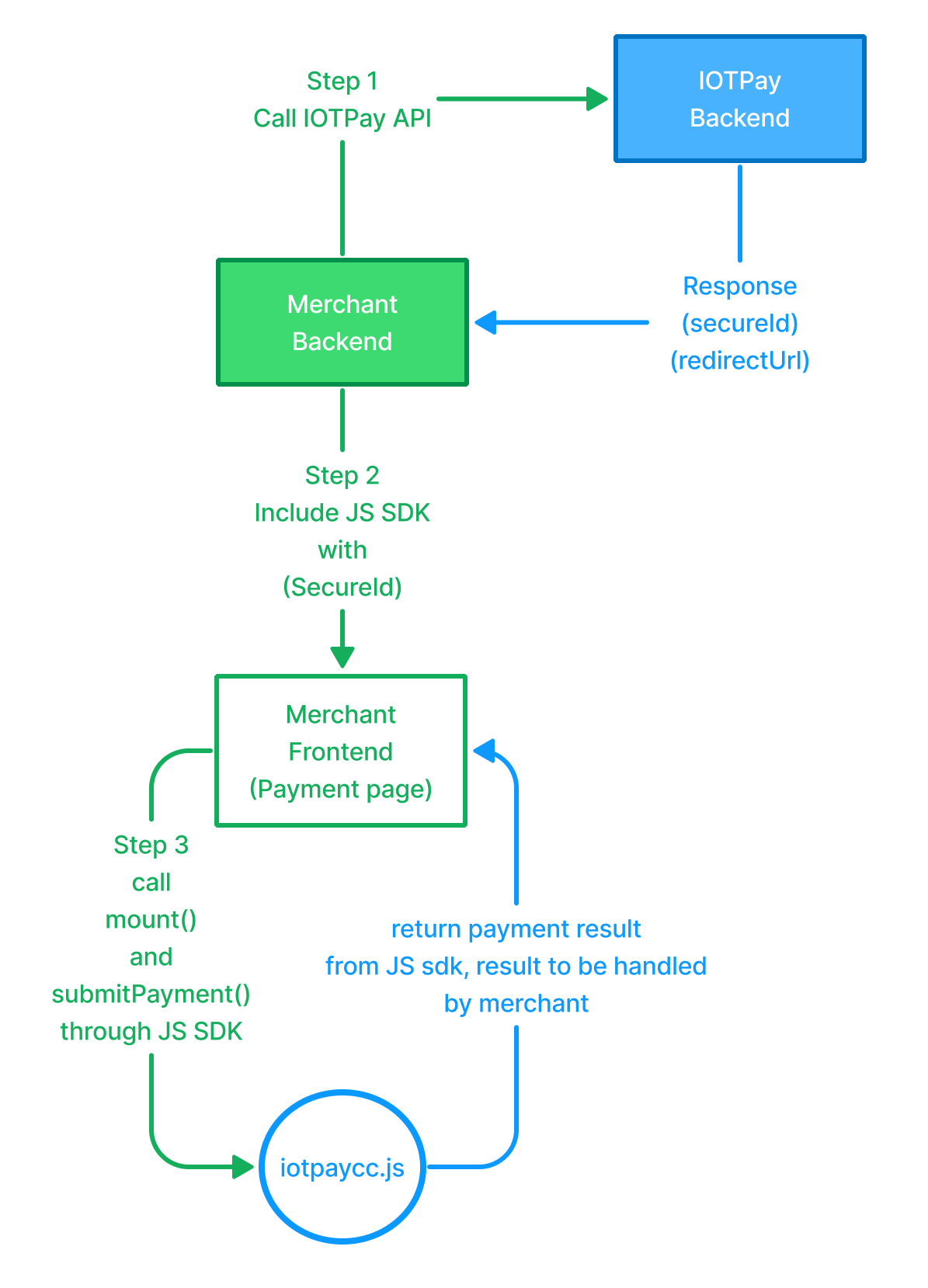
Backend Integration
Info
Make sure that requests to IOTPay endpoints are performed on the merchant's server (as opposed to the client side browser) to avoid potential CORS errors.
Card Purchase
Make a POST request to the cc_purchase
API endpoint on your backend server.
Endpoint
https://ccapi.iotpaycloud.com/v3/cc_purchase
Method
POST
Header
Content-Type: application/json;charset=UTF-8
Request
name | required | type | sample | description |
---|---|---|---|---|
mchId | y | String(30) | 10000701 | assigned by IOTPay |
mchOrderNo | y | String(30) | 1234567890abc | assigned by merchant |
amount | y | Int | 1500 | in cents |
currency | y | String(3) | CAD | for now only CAD supported |
loginName | y | String(12) | jack123 | merchant's login name |
subject | n | String(64) | ||
body | n | String(250) | ||
channel | y | String | PF_CC | PF_CC or UPI_EX |
notifyUrl | y | String(200) | get notify when success | |
returnUrl | y | String(200) | https://example.com | redirect to this url after payment |
sign | y | String(32) | C380BEC2BFD727A4B6845133519F3AD6 | Sign algorithm |
Response
name | required | type | sample | description |
---|---|---|---|---|
retCode | y | String | SUCCESS or FAIL | |
retMsg | y | String | ||
retData.redirectUrl | y | String | For redirect purchase, please ignore this field for embedded integration | |
retData.secureId | y | String | For embedded SDK integration only |
Info
Make the secureId available to the client.
Card Tokenize
Make a POST request to the cc_addCard
API endpoint on your backend server.
Endpoint - Add Card
https://ccapi.iotpaycloud.com/v3/cc_addCard
Method
POST
Header
Content-Type: application/json;charset=UTF-8
Request
Name | Required | Type | Sample | Description |
---|---|---|---|---|
mchId | y | String(30) | 0000701 | assigned by IOTPay |
cardId | y | String(30) | 604567999 | assigned by merchant, must be unique |
loginName | y | String(12) | jack123 | merchant's login name |
channel | y | String | PF_CC | PF_CC or UPI_EX |
returnUrl | y | String(200) | redirect to this url after card is added | |
sign | y | String(32) | C380BEC2BFD727A4B6845133519F3AD6 | Sign algorithm |
Please note
Each cardId
can bind only one credit card, if one user need to bind more cards, use different cardId
Response
name | required | type | sample | description |
---|---|---|---|---|
retCode | y | String | SUCCESS or FAIL | |
retMsg | y | String | ||
retData.redirectUrl | y | String | For redirect purchase, please ignore this field for embedded integration | |
retData.secureId | y | String | For embedded SDK integration only | |
retData.channel | y | String | PF_CC | PF_CC or UPI_EX |
Info
Make the secureId available to the client.
Frontend Integration
Info
You will need the secureId
from response above to create a IOTPay iframe instance through JS SDK.
Add a placeholder div
<div id="iotpay_normal"></div>
Add a html script
tag
<script
type="text/javascript"
src="https://ccapi.iotpaycloud.com/cc/iotpaycc.js"
></script>
const script = document.createElement('script')
script.src = 'https://ccapi.iotpaycloud.com/cc/iotpaycc.js'
script.type = 'text/javascript'
script.async = true
Once you received the secureId from the API call, mount the iframe in the place holder div
WARNING
To be PCI compliant, you must load IOTPay.js directly from https://ccapi.iotpaycloud.com/cc/iotpaycc.js. You cannot include it in a bundle or host it yourself.
<script>
let callback = function (event) {
console.log(event)
if (event.result == 'SUCCESS') {
// Add/Pay Success
// Return data will be contained in event.detail
// if (event.detail.retData && event.detail.retData.redirectUrl) {
// window.location.replace(event.detail.retData.redirectUrl);
// }
} else if (event.result == 'FAIL' && event.message == 'Timeout') {
// Union Pay require query orders. We will be timed out after 30 tries and merchant needs to query the order.
//
} else if (event.result == 'FAIL') {
// Add/Pay Failed
// Return data will be contained in event.detail
// if (event.detail.retData && event.detail.retData.redirectUrl) {
// window.location.replace(event.detail.retData.redirectUrl);
// }
}
if (event.detail.retData && event.detail.retData.redirectUrl) {
window.location.replace(event.detail.retData.redirectUrl)
}
}
let secureId = '3abcd*******3abcd' //get secureId from addCard or Purchase endpoint.
let iotpay_normal = Iotpay(secureId, 'Pay') // second params must be Add (Add card) or Pay
iotpay_normal.mount('#iotpay_normal', callback)
</script>
async loadIOTLibrary() {
const script = document.createElement('script');
script.src = "https://ccapi.iotpaycloud.com/cc/iotpaycc.js";
script.type = "text/javascript";
script.async = true;
return new Promise((resolve, reject) => {
script.onload = () => {
// First, we need to call API to get the secureId
let reqBody = {
mchUserId: "test",
mchOrderNo: uuidV4(),
amount: this.state.order.total * 100, // make sure the unit is in cents
channel: "PF_CC", // or UPI_EX
returnUrl: "https://test.merchant.com/payment/result",
notifyUrl: "https://test.merchant.com/webhook/getNotify"
}
// Replace the second parameter with merchant's API key
reqBody = this.getSignedBody(reqBody, "==== merchantAPIKey ====");
// A request to merchant's backend server, where it would make a call to 'cc_purchase'
axios.post('http://test.merchant.server/v1/iotpay/purchase', reqBody)
.then(res => {
if (res.status === 200 && res.data.retCode === "SUCCESS" && res.data.retData.secureId) {
let order = this.state.order;
order.payment_id = res.data.retData.secureId;
this.setState({ order })
// iframe options, this field is not mandatory
const options = {
"darkMode": true,
"theme": "card",
"button": {
"text": "Pay Now!",
"color": "#fff",
"backgroundColor": "#f90",
"backgroundImage": "none",
"boxShadow": "none",
"height": "60px",
"borderRadius": '0px'
}
};
// second params must be Add (Add a customer creditCard for future purchase) or Pay
let iotpay_normal = window.Iotpay(res.data.retData.secureId, 'Pay', options);
// IOTCallback is a function that handles payment result callbacks
iotpay_normal.mount('#iotpay_normal', this.IOTCallback);
} else {
// handle failed request - example:
reject(new Error(res.data.retMsg))
}
})
resolve();
}
script.onerror = () => {
reject(new Error('Failed to load IOTPay library.'));
};
document.body.appendChild(script);
})
}
IOTCallback(event) {
console.log(event);
if (event.result === 'SUCCESS') {
// Add/Pay Success
// Return data will be contained in event.detail
// if (event.detail.retData && event.detail.retData.redirectUrl) {
// window.location.replace(event.detail.retData.redirectUrl);
// }
} else if (event.result === 'FAIL' && event.message === 'Timeout') {
// Union Pay require query orders. We will be timed out after 30 tries and merchant needs to query the order.
//
} else if (event.result === 'FAIL') {
// Add/Pay Failed
// Return data will be contained in event.detail
// if (event.detail.retData && event.detail.retData.redirectUrl) {
// window.location.replace(event.detail.retData.redirectUrl);
// }
}
if (event.detail.retData && event.detail.retData.redirectUrl) {
window.location.replace(event.detail.retData.redirectUrl);
}
}
Additional SDK and Documents
For iOS integration: iOS sdk
For Android integration: Android sdk
PHP and JS integration: Php sdk